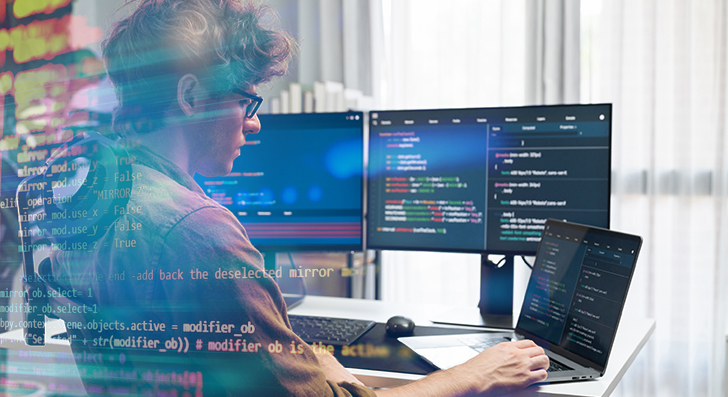
Scalability means your application can cope with progress—a lot more customers, extra facts, and a lot more site visitors—with out breaking. As a developer, making with scalability in mind will save time and anxiety later. Below’s a clear and simple guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be portion of your system from the beginning. Quite a few applications fall short when they increase rapid since the first style can’t cope with the extra load. For a developer, you have to Assume early about how your procedure will behave under pressure.
Start out by creating your architecture being adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out influencing the whole program.
Also, contemplate your databases from day 1. Will it need to have to take care of a million consumers or just a hundred? Choose the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them but.
A different vital issue is to avoid hardcoding assumptions. Don’t write code that only functions below existing problems. Contemplate what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or party-pushed programs. These support your app manage a lot more requests without having obtaining overloaded.
Whenever you Develop with scalability in mind, you're not just making ready for fulfillment—you happen to be minimizing potential head aches. A well-prepared process is simpler to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is really a crucial part of setting up scalable apps. Not all databases are constructed the same, and utilizing the Improper you can sluggish you down or perhaps induce failures as your application grows.
Start off by comprehending your details. Could it be extremely structured, like rows inside of a table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are definitely sturdy with relationships, transactions, and consistency. They also aid scaling strategies like browse replicas, indexing, and partitioning to deal with more targeted traffic and data.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing huge volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and create designs. Are you carrying out numerous reads with less writes? Use caching and browse replicas. Are you presently handling a weighty produce load? Look into databases that will cope with high create throughput, as well as celebration-primarily based knowledge storage devices like Apache Kafka (for non permanent info streams).
It’s also sensible to Imagine in advance. You might not require Superior scaling characteristics now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based upon your obtain patterns. And often check database efficiency while you expand.
In a nutshell, the correct database is determined by your app’s structure, pace wants, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is vital to scalability. As your app grows, every compact hold off provides up. Badly composed code or unoptimized queries can slow down performance and overload your system. That’s why it’s imperative that you Make economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Option if an easy a single functions. Keep the features short, centered, and easy to check. Use profiling resources to find bottlenecks—spots exactly where your code usually takes way too prolonged to run or employs an excessive amount of memory.
Future, have a look at your databases queries. These typically gradual factors down more than the code by itself. Make sure Every single query only asks for the info you really have to have. Prevent SELECT *, which fetches anything, and rather pick out specific fields. Use indexes to speed up lookups. And stay clear of performing a lot of joins, Specifically throughout large tables.
In case you see exactly the same facts being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached therefore you don’t have to repeat pricey get more info functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to exam with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when wanted. These ways assistance your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to take care of more users and more visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, stable, and scalable.
Load balancing spreads incoming visitors across numerous servers. Rather than one server accomplishing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can mail visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When people request the same facts once again—like a product website page or maybe a profile—you don’t must fetch it from the databases each and every time. You can provide it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops facts in memory for quickly obtain.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t modify normally. And generally make certain your cache is up-to-date when details does modify.
Briefly, load balancing and caching are easy but strong applications. With each other, they assist your application handle a lot more buyers, stay quickly, and Get well from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need instruments that permit your application develop very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t really have to buy hardware or guess long term capacity. When visitors raises, you'll be able to incorporate far more means with just some clicks or automatically using vehicle-scaling. When targeted visitors drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may target constructing your app rather than managing infrastructure.
Containers are another vital tool. A container offers your application and every little thing it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 section of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale areas independently, that is great for effectiveness and reliability.
To put it briefly, employing cloud and container tools signifies you can scale rapidly, deploy effortlessly, and Get well quickly when troubles happen. If you need your application to expand without the need of limits, start out using these equipment early. They conserve time, lessen risk, and enable you to keep centered on creating, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make greater decisions as your app grows. It’s a essential Component of building scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over how long it will require for buyers to load internet pages, how frequently glitches materialize, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This allows you deal with difficulties speedy, generally ahead of people even observe.
Monitoring is also practical any time you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works effectively, even stressed.
Last Views
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Get started tiny, Assume big, and Construct clever.